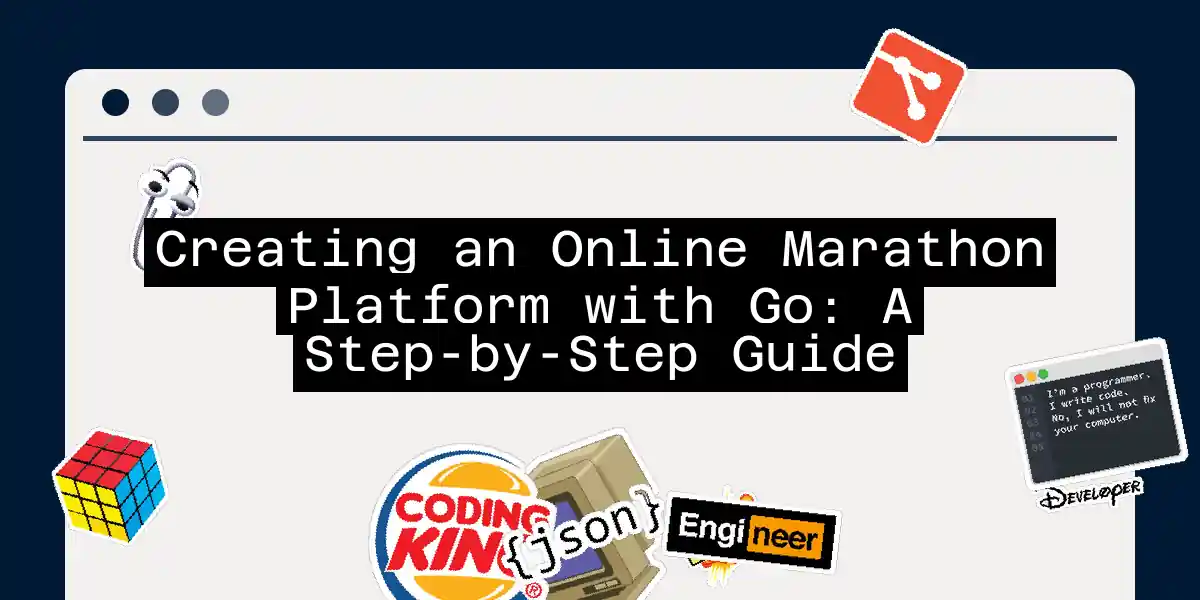
Creating an Online Marathon Platform with Go: A Step-by-Step Guide
Introduction to Go and Its Benefits Before diving into the nitty-gritty of creating an online marathon platform, let’s briefly discuss why Go (also known as Golang) is an excellent choice for this task. Go, developed by Google, is designed to handle the challenges of building scalable and high-performance applications. Here are some key benefits that make Go a great fit for our project: High Performance: Go compiles to machine code, ensuring fast execution speeds. This is crucial for handling a large number of concurrent requests, which is typical in online marathon platforms. Simple Syntax: Go’s minimalist syntax makes it easy to learn and use, reducing the likelihood of errors and allowing developers to start coding quickly. Built-in Concurrency Support: Go provides convenient mechanisms for handling parallel tasks, such as goroutines and channels, which are essential for managing multiple simultaneous requests. Static Typing: Go’s strict type system helps avoid many errors at the compilation stage, making the code more reliable and easier to maintain. Setting Up the Environment To start developing your online marathon platform, you need to set up your Go environment. Here are the steps: ...